How to Create Meme Coin on Solana Network
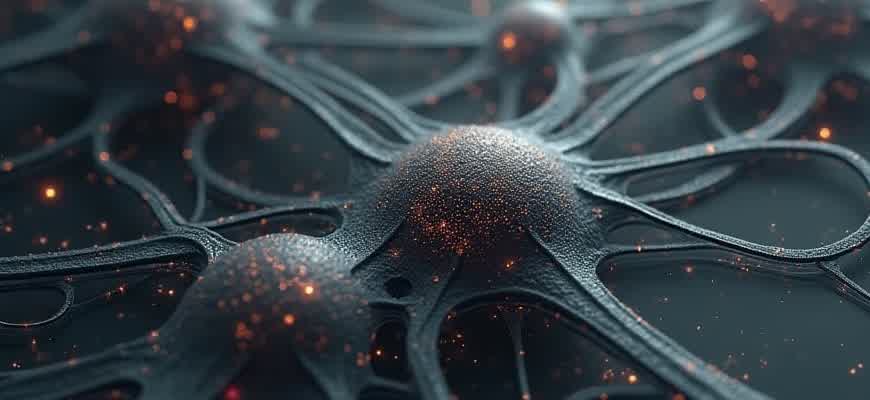
Creating a meme cryptocurrency on the Solana network is a process that involves multiple steps, from setting up your development environment to deploying your token. Below is a simplified guide on how to create your own meme coin using Solana's blockchain.
Before diving into the creation of your token, ensure you have the necessary tools and knowledge to interact with the Solana network. Below is a checklist of requirements:
- Solana wallet (e.g., Phantom or Sollet)
- Solana CLI (Command Line Interface) installed on your machine
- Rust programming language knowledge (for custom token logic)
- Access to Solana’s testnet or devnet for testing purposes
Tip: Familiarize yourself with Solana’s token program to understand how tokens are issued and managed on the network.
The process of creating your meme coin involves several key steps:
- Set up your development environment: Install Solana CLI and create a wallet to interact with the blockchain.
- Create a token: Using Solana’s Token Program, you can create a new token using the “spl-token” command-line tool.
- Deploy your token: After creating your meme coin, deploy it to Solana’s mainnet (or testnet if you prefer testing first).
Here’s a table summarizing the essential commands:
Action | Command |
---|---|
Install Solana CLI | curl -sSf https://release.solana.com/stable/install | sh |
Create Token | spl-token create-token |
Create Token Account | spl-token create-account |
Reminder: Always test your meme coin on the Solana devnet before deploying it on the mainnet to avoid any costly mistakes.
Setting Up Your Development Environment for Solana
To create a meme coin on the Solana blockchain, you need to first set up a suitable development environment. This includes installing various tools and dependencies that will allow you to interact with the Solana network and deploy your token. Below are the essential steps to get started.
Before diving into coding, ensure that you have the right set of tools and dependencies installed. This will include the Solana CLI, Rust programming language, and related libraries that facilitate smart contract development on Solana.
1. Install Required Tools
- Solana CLI - The Solana command-line interface (CLI) is essential for managing keypairs, sending transactions, and interacting with the Solana network.
- Rust - Rust is the primary programming language for writing smart contracts on Solana (known as "programs"). Ensure you have the latest version of Rust installed.
- Anchor Framework - Anchor is a framework built on top of Solana that simplifies the development of smart contracts.
2. Step-by-Step Installation
- Install Solana CLI: Run the following command to install Solana CLI on your system:
curl --proto '=https' --tlsv1.2 -sSf https://release.solana.com/v1.10.32/install
- Install Rust: Use Rust’s official installation tool (rustup) by running:
curl --proto '=https' --tlsv1.2 -sSf https://sh.rustup.rs | sh
- Install Anchor: Anchor is crucial for simplifying contract creation and deployment. Install it by running:
cargo install --git https://github.com/project-serum/anchor anchor-cli --locked
3. Configuration
After installation, configure your environment to connect to Solana’s devnet or testnet for safe development and testing.
Important: Always test your coin or smart contract on a test network (devnet/testnet) before deploying to the mainnet to avoid unnecessary losses.
4. Verifying the Setup
Once the tools are installed and configured, verify that everything is working correctly by running a few simple commands.
Command | Purpose |
---|---|
solana --version | Check the installed version of the Solana CLI |
rustc --version | Ensure Rust is properly installed |
anchor --version | Verify Anchor is installed |
Choosing the Right Token Standards for Your Meme Coin
When creating a meme coin on the Solana network, selecting the appropriate token standard is a crucial decision. The Solana blockchain supports several token standards, each with its own set of features and functionalities. Understanding these standards will allow you to choose one that aligns best with your project's objectives, whether you're aiming for a simple token, a community-driven initiative, or a more complex ecosystem.
Two primary token standards on Solana are the SPL Token and the SPL Token with custom metadata. Each comes with its benefits and limitations. To make an informed choice, you need to assess your specific requirements and how they match the characteristics of these token standards.
SPL Token
The SPL Token is the most widely used standard on Solana. It’s known for being lightweight and highly efficient, making it a suitable choice for most basic meme coin projects.
- Low transaction fees: Transactions involving SPL tokens are very cost-effective.
- Fast processing times: Solana's scalability ensures that token transfers are executed rapidly.
- Wide compatibility: SPL tokens are compatible with a large number of wallets and platforms built on Solana.
Custom SPL Token with Metadata
If your meme coin involves more advanced features, such as detailed community interaction, token attributes, or complex reward mechanisms, you may opt for a custom SPL Token with additional metadata.
- Enhanced features: This allows you to integrate specific properties like image URLs, descriptions, and additional data.
- Community engagement: With custom metadata, users can interact with tokens in more meaningful ways, such as displaying meme-related media.
- Flexibility: Provides room for future updates and feature expansion, such as governance tokens or staking functionality.
Choosing between a standard SPL token and one with custom metadata largely depends on your meme coin’s goals. For simple and low-cost coins, the SPL Token is ideal. However, for projects that require more engagement and flexibility, incorporating metadata might be the best choice.
Key Considerations
Here’s a comparison to help you choose the right standard for your meme coin:
Feature | SPL Token | Custom SPL Token with Metadata |
---|---|---|
Transaction Fees | Low | Low |
Speed | High | High |
Customization | Limited | Extensive |
Use Case | Basic coins | Community engagement, collectibles |
Writing the Smart Contract for Your Meme Coin
Creating a meme coin on the Solana network involves designing a smart contract that will control the behavior of your token. The smart contract is responsible for defining the coin’s issuance, transfers, and other functionalities. Solana's smart contract programming is typically done using Rust or C, which allows for high efficiency and scalability on the network.
When writing a smart contract for your meme coin, you'll first need to decide on the core features and properties of your token. These could include the token's name, symbol, supply limit, and decimal precision. Additionally, you’ll define the rules for minting, transferring, and burning tokens. The smart contract code must ensure that all operations happen securely and in accordance with your design.
Key Considerations for Writing the Contract
- Token Name and Symbol: Choose a unique name and symbol for your meme coin. These will be used by wallets and exchanges to identify your token.
- Total Supply: Define the total amount of tokens available in the ecosystem. This will impact scarcity and the potential value of your meme coin.
- Decimals: Set the decimal precision for your token. This determines how divisible your meme coin can be.
- Minting and Burning: Decide if users or a central authority can mint or burn tokens. This will help control the supply and inflation of your meme coin.
Steps to Build the Smart Contract
- Set up your development environment with the necessary Solana SDK and Rust/C libraries.
- Define the structure of your token contract using the Solana token program, including key attributes like minting, transfers, and the owner.
- Write functions to handle token transfers, checking balances, and other functionalities like freezing accounts or pausing operations if needed.
- Deploy the contract to the Solana testnet for testing before moving to the mainnet.
- Ensure the contract’s security by performing audits and running tests to prevent any vulnerabilities.
Note: Proper testing is crucial before deploying your smart contract on the Solana mainnet. Any errors in the contract can result in lost funds or security risks.
Smart Contract Overview
Function | Description |
---|---|
Initialize | Sets up the initial parameters for your meme coin, such as supply and owner address. |
Transfer | Allows the transfer of tokens from one account to another. |
Mint | Creates new tokens and adds them to the circulating supply. |
Burn | Destroys tokens, reducing the total supply. |
Deploying Your Meme Coin on the Solana Network
Once you've completed the development of your meme coin, it's time to deploy it on the Solana blockchain. This step involves transferring your code to the network and ensuring it's publicly accessible for transactions. Solana's fast transaction processing and low fees make it an attractive choice for launching tokens. However, deploying requires a few technical steps that you need to follow carefully.
In this guide, we'll cover the necessary steps to deploy your meme coin on the Solana Network using the Rust programming language and the Solana CLI tool. You’ll also need to have a funded wallet to pay for transaction fees during the deployment process.
Steps for Deployment
- Set up the Solana CLI: First, install the Solana CLI tool on your computer. This tool allows you to interact with the Solana blockchain from your terminal. You can download it from the official Solana website.
- Create a Wallet: You’ll need to create a wallet on the Solana network to handle the tokens and cover the deployment costs. Use the Solana CLI to generate a new wallet, then fund it with SOL tokens.
- Deploy Token Program: Solana uses a specific program for token creation. Deploy this program to the network by running the necessary commands from the Solana CLI.
- Mint the Tokens: After the program is deployed, mint the tokens using the token minting commands in the CLI. This creates the initial supply of your meme coin.
- Verify Deployment: Once the deployment is complete, verify that your meme coin is live on the Solana blockchain by checking the token's details using the Solana Explorer.
Important Considerations
Security: Always ensure that your wallet and private keys are secured. Losing your keys means losing access to your tokens.
Step | Description |
---|---|
Install Solana CLI | Download and install Solana CLI from the official Solana site. |
Create Wallet | Use CLI to generate and fund a wallet for deployment. |
Deploy Token Program | Deploy the token creation program using the Solana CLI. |
Mint Tokens | Create the initial supply of your meme coin. |
Verify | Ensure the coin is live by checking the blockchain explorer. |
Minting Your Meme Coins: Step-by-Step Guide
Minting a meme coin on the Solana blockchain involves several critical steps that require some basic understanding of Solana's tools and the technical requirements for creating and deploying tokens. With Solana's high throughput and low transaction fees, it's an ideal platform for launching meme coins, offering the flexibility and scalability needed for such projects. This guide will walk you through the entire process, from setting up your wallet to deploying the coin on the Solana network.
The process of minting a meme coin involves creating a new token on Solana, setting its properties, and then distributing or managing it. You will need a Solana wallet, some SOL tokens to cover transaction fees, and a command-line interface (CLI) tool for creating and deploying the coin. Here’s how you can go about it.
1. Setting Up Your Environment
- Install Solana CLI: You need to install the Solana Command Line Interface (CLI) tool, which is essential for interacting with the blockchain. Use the official guide for installation.
- Set up a wallet: You will need a Solana wallet to store your SOL tokens and manage your meme coin. Popular options include Phantom and Sollet wallets.
- Fund your wallet: Transfer some SOL to your wallet to cover the costs of minting and other transactions.
2. Creating the Meme Coin
- Use the Solana Token Program: This is a smart contract used for creating custom tokens on the network. The Token Program provides the basic functionality you need.
- Run the minting command: Once the CLI is set up, run the minting command to create a new token. Make sure to define the token name, symbol, and initial supply.
- Verify the token creation: After minting, verify the token by checking its details using the CLI tool.
3. Token Management
Action | Command |
---|---|
Mint Additional Tokens | solana-tokens mint token-address amount |
Transfer Tokens | solana-tokens transfer recipient-address amount |
Freeze Token Account | solana-tokens freeze account-address |
Important: Be sure to keep your private keys and wallet recovery phrases safe. Losing access to these could result in the loss of your meme coin project.
4. Deploying and Distributing the Coin
- Distribute the meme coins by transferring them to wallets of your users or community members. You can integrate your token with existing platforms, such as decentralized exchanges (DEXs), for trading.
- To make the coin available for others, consider listing it on decentralized exchanges or building a website for your meme coin.
How to List Your Meme Coin on Decentralized Exchanges
Once you have successfully created your meme coin on the Solana network, the next crucial step is to list it on decentralized exchanges (DEXs). Listing on DEXs helps provide liquidity and allows your community to easily trade the coin. While Solana's fast transaction times and low fees make it an ideal blockchain for meme coins, the process of getting your coin on an exchange requires several steps that should be approached with care and preparation.
In this process, you'll need to ensure your coin meets the necessary requirements for listing, select appropriate platforms, and promote your coin effectively to drive trading activity. Here’s a step-by-step guide to help you navigate the process of listing your meme coin on decentralized exchanges.
Step-by-Step Process
- Prepare Your Coin's Token Details
- Token name
- Token symbol
- Supply and distribution details
- Smart contract address
- Choose the Right Decentralized Exchanges
Ensure that the DEX you are considering supports Solana-based tokens, such as Serum or Raydium. These platforms are well-integrated with Solana’s ecosystem and ensure faster transactions.
- Submit Your Coin for Listing
Each DEX has its own listing process. Generally, you will need to submit an application and provide necessary documentation, such as a whitepaper, tokenomics, and security audit results if applicable.
- Provide Liquidity
- Provide initial liquidity to your coin on the exchange to allow users to trade it.
- Ensure enough liquidity in the pool to prevent price manipulation and maintain a stable market.
Important Information
Listing on decentralized exchanges is often free but can require an active community to ensure liquidity and successful trading. It's essential to engage your community and ensure continuous growth after listing.
Key Metrics
Metric | Importance |
---|---|
Community Engagement | High - A strong community supports liquidity and demand. |
Initial Liquidity | High - Without liquidity, your coin will be difficult to trade. |
Tokenomics | High - Clear and transparent tokenomics build trust and credibility. |
Building a Community Around Your Meme Coin
Creating a strong and engaged community is essential for the success of your meme coin. People are more likely to invest and participate in a coin that has an active and passionate following. The key to building a vibrant community lies in consistent interaction, clear communication, and providing incentives that keep members involved and excited about your project.
There are several methods to engage potential users and create lasting relationships. The foundation of a successful community is trust and transparency, which can be fostered through regular updates, open discussions, and the creation of meaningful partnerships.
Strategies for Community Growth
- Leverage Social Media: Platforms like Twitter, Reddit, and Telegram are ideal for sharing updates, interacting with users, and generating hype around your coin. Make sure to engage with community members regularly.
- Create a Dedicated Website: A well-designed website provides a hub for information about your project. Include a roadmap, FAQs, and an active blog to keep people informed.
- Host Giveaways and Contests: Incentives like token giveaways or meme creation contests encourage participation and attract new users.
Engagement Tools
- Telegram Groups: Set up dedicated channels for discussions and updates. This allows for direct interaction with your community.
- AMA Sessions: Hold regular "Ask Me Anything" sessions where users can ask questions and learn more about the project.
- Influencer Collaborations: Partner with crypto influencers to expand your reach and gain credibility.
Building a community isn't just about numbers–it's about creating an environment where users feel valued, heard, and excited to contribute to your project's success.
Community Engagement Metrics
Metric | Importance |
---|---|
Active Social Media Followers | Measures the reach and engagement of your content. |
Telegram Group Activity | Indicates the level of interest and participation in discussions. |
Participation in Giveaways | Shows how effective your incentives are in driving engagement. |
Managing the Long-Term Success of Your Meme Coin
Maintaining the growth and sustainability of a meme coin involves more than just initial hype. It requires a strategic approach that focuses on community engagement, consistent development, and long-term value creation. A successful meme coin not only attracts investors but keeps them engaged over time through innovation and utility.
To ensure the longevity of your meme coin, it's crucial to focus on these key aspects:
1. Community Building and Engagement
One of the pillars of a successful meme coin is its community. Without an engaged and active group of supporters, the coin's value will quickly diminish. Here's how you can manage this:
- Frequent Communication: Regular updates on social media and community platforms are essential to keep users informed.
- Exclusive Rewards: Offer unique incentives like airdrops or giveaways to encourage ongoing participation.
- Active Moderation: Ensure that your community space remains welcoming and productive to foster positive interaction.
2. Innovation and Use Cases
Developing use cases and real-world applications for your meme coin can set it apart from others in the market. This keeps the coin valuable beyond just speculation. Consider these strategies:
- Partnerships: Collaborate with other projects or platforms to provide additional utility to the coin.
- Develop New Features: Continuously improve the coin's features and ecosystem to address evolving market needs.
- Integration: Aim for the coin to be accepted for purchases or services within certain ecosystems.
3. Maintaining Transparency and Trust
Trust is the foundation of any cryptocurrency’s long-term success. Without transparency, your project risks losing credibility. Key actions include:
Always maintain transparency about your project's progress, challenges, and future plans. Open communication builds trust with your community and potential investors.
4. Financial Management and Security
Financial stability is crucial for long-term sustainability. Managing funds and ensuring the security of your project should be a top priority:
Action | Description |
---|---|
Liquidity Management | Ensure there’s enough liquidity for users to trade without significant slippage. |
Security Audits | Regularly audit the smart contracts and platforms associated with your meme coin to avoid hacks or vulnerabilities. |