Create Meme Coin on Eth
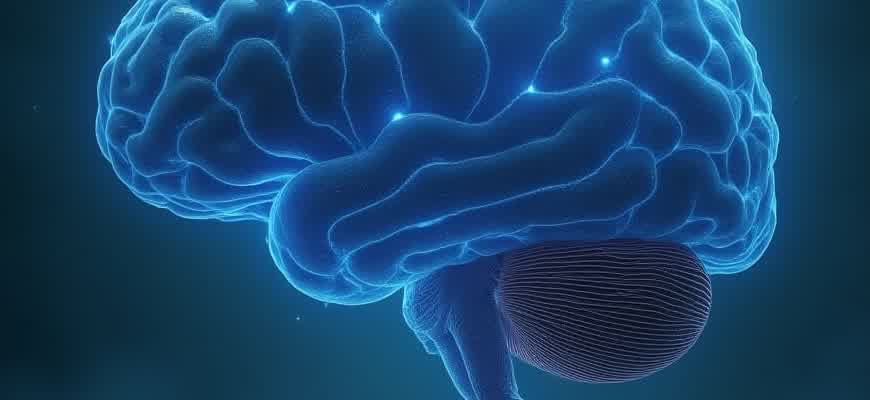
Creating a meme token on Ethereum is an exciting way to tap into the growing interest in digital currencies, leveraging both the blockchain's security and decentralized nature. The process involves creating a custom token, deploying it to the network, and ensuring it gains attention in the right communities. Here's how you can get started:
- Define the purpose of the meme token and its potential use case.
- Write the smart contract for the token using Solidity.
- Deploy the contract on the Ethereum network using a platform like Remix or Truffle.
- Market your token to relevant online communities and influencers.
Key Considerations:
Creating a successful meme coin requires more than just coding. Building a community and understanding the cultural trends are essential for the meme's growth and success.
The next step is to write the smart contract for the token. Most meme tokens on Ethereum use the ERC-20 standard, which provides the basic functionality for creating a tradable asset. The code must include functions like transferring tokens, querying balances, and approving transactions. A well-written contract ensures the token operates smoothly and securely.
Function | Description |
---|---|
transfer() | Allows users to send tokens to another address. |
balanceOf() | Returns the token balance of an address. |
approve() | Lets a user approve another address to spend their tokens on their behalf. |
How to Select a Memecoin Idea for Your Ethereum Token
Creating a successful memecoin on Ethereum involves more than just technical execution–it’s about selecting the right concept that resonates with the community and reflects current trends. Memecoins thrive on internet culture, so understanding what captures attention and fuels engagement is essential. When choosing an idea for your token, it’s crucial to keep in mind the target audience and the long-term potential for growth. The goal is not just to create hype, but to generate a loyal user base who are motivated by fun, humor, and memes that align with the token’s theme.
At the core of any memecoin is its concept. A great idea can turn an obscure token into a viral sensation. Memecoin concepts can range from animal-themed tokens to pop culture references, but the concept needs to be unique enough to stand out. It must also be easily relatable and sharable, as memecoins heavily rely on social media engagement for growth.
Steps to Choose a Memecoin Concept
- Consider Current Trends: Look at what’s popular in the meme space right now. Is there a viral challenge, trend, or meme format that could be transformed into a token idea? Keeping up with the latest internet phenomena is key to ensuring your coin gets noticed.
- Pick a Strong Symbol or Mascot: Memecoins often thrive around recognizable figures or animals. For example, Dogecoin became popular due to the Shiba Inu dog. Your token should have a memorable and fun symbol that makes it easy for people to relate to.
- Community Involvement: Engage with your potential community early. What are people talking about on Twitter, Reddit, or other social platforms? Creating a concept based on their interests can help you build an active community.
Key Factors to Keep in Mind
- Humor and Entertainment Value: The primary driver of most successful memecoins is humor. A funny concept can capture the attention of a wide audience.
- Memorability: People are more likely to invest in a coin that sticks in their minds. A clever name, an iconic logo, or a funny storyline can help your token stand out.
- Scalability: Your memecoin should have the potential to grow and evolve. Choose a concept that can be expanded on through new memes, partnerships, or events.
Example Concepts
Concept | Description |
---|---|
Animal-themed Tokens | Tokens based on animals like dogs, cats, or even mythical creatures can resonate with pet owners or animal lovers. |
Pop Culture References | Create tokens inspired by movies, celebrities, or trends that are currently trending in pop culture. |
Crypto Meme Characters | Characters or phrases from within the crypto community itself, like “HODL” or “to the moon,” could form the basis for a memecoin. |
Tip: Choose a concept that not only speaks to your audience but also has the potential for virality. The more shareable, the better.
Setting Up Your Development Environment for Ethereum-based Tokens
Before diving into token creation on the Ethereum network, it's crucial to set up a development environment that allows smooth interaction with the blockchain. This involves installing necessary tools, setting up a local test network, and ensuring that your development system is prepared for coding smart contracts. Here’s a step-by-step guide to setting things up.
The main components required for developing Ethereum-based tokens include a development framework, a local Ethereum node, and a code editor. The most popular tools for Ethereum smart contract development include Truffle, Hardhat, and Remix IDE. Additionally, you’ll need Node.js and npm to manage packages and dependencies. Once your environment is properly configured, you can start coding and testing your token contract before deploying it to the live Ethereum network.
Essential Tools for Development
- Node.js - A JavaScript runtime that lets you run code outside of a browser.
- npm - A package manager for JavaScript, essential for managing dependencies.
- Truffle or Hardhat - Development frameworks for writing, testing, and deploying smart contracts.
- Ganache - A personal Ethereum blockchain for testing contracts locally.
- MetaMask - A browser extension to interact with the Ethereum network via your wallet.
- Solidity - The primary programming language for writing smart contracts on Ethereum.
Installation Steps
- Install Node.js and npm: Download and install the latest version of Node.js from the official website. This will automatically install npm.
- Install Truffle/Hardhat: Run the following command to install Truffle globally:
npm install -g truffle
or install Hardhat with:
npm install --save-dev hardhat
- Set up a local Ethereum blockchain: Use Ganache or Hardhat Network to create a personal Ethereum blockchain for testing your token contract.
- Install MetaMask: Add MetaMask to your browser and configure it to connect to your local blockchain or the Ethereum test network.
Blockchain Testing and Deployment
Before deploying your token to the main Ethereum network, it’s essential to test it thoroughly. You can use either Ganache or a public testnet like Rinkeby or Goerli to test your contract. Once your contract is deployed on the test network and functions as expected, you can proceed with the deployment to the Ethereum mainnet.
Tip: Always test your contract on a testnet to avoid spending real ETH on transactions during development and testing.
Sample Setup Table
Tool | Description | Installation Command |
---|---|---|
Node.js | JavaScript runtime for Ethereum development | Download from nodejs.org |
Truffle | Development framework for Ethereum | npm install -g truffle |
Ganache | Local Ethereum blockchain for testing | Download from trufflesuite.com/ganache |
MetaMask | Ethereum wallet extension for browsers | Install from metamask.io |
Writing the Smart Contract for Your Meme Coin
Creating the smart contract for your meme coin involves defining its key features, such as total supply, minting, and token distribution. The smart contract is the backbone of your token’s functionality on the Ethereum blockchain, which ensures transparency and automation of all transactions. It’s essential to plan the structure of the token before diving into the coding process.
The primary steps in writing the contract are understanding the ERC-20 standard, writing the contract code, deploying it to the Ethereum network, and testing the contract thoroughly. These stages ensure that your token will be fully functional and secure. Below are the critical components to include in your smart contract:
Key Elements of the Smart Contract
- Token Name and Symbol: Defines the name and abbreviation of your meme coin (e.g., "MemeCoin" with symbol "MEME").
- Total Supply: Determines the total number of tokens that will ever exist.
- Decimals: Defines how divisible the coin will be, typically set to 18.
- Minting and Burning: Rules on how tokens are created or destroyed, ensuring flexibility in token supply.
- Ownership: A designated address with administrative rights, such as contract updates or freezing transfers in case of an emergency.
Steps to Writing the Contract
- Define Token Parameters: Write the basic structure of your token, including name, symbol, total supply, and decimals.
- Implement ERC-20 Functions: Use standard functions like transfer(), balanceOf(), approve(), and transferFrom() to ensure compatibility with the Ethereum network.
- Test the Contract: Run the contract on a test network to check for any vulnerabilities or issues.
- Deploy the Contract: Once the code is verified, deploy the contract to the Ethereum mainnet.
Important: Always conduct an audit or use security tools before deploying the smart contract to prevent vulnerabilities, as once deployed, it cannot be changed.
Example of a Simple ERC-20 Token Smart Contract
Function | Purpose |
---|---|
totalSupply() | Returns the total supply of the tokens. |
balanceOf(address) | Checks the balance of tokens in a specific address. |
transfer(address, uint) | Transfers tokens from one address to another. |
Deploying Your Memecoin Smart Contract on the Ethereum Network
Once you've developed your memecoin smart contract, the next step is to deploy it to the Ethereum blockchain. Deployment involves creating a transaction that places your contract on the network, allowing users to interact with it. This process requires knowledge of smart contract deployment tools and understanding of the Ethereum network’s gas fees.
The deployment process can be divided into several key steps. Before proceeding, ensure your contract code is fully tested and audited to avoid vulnerabilities and errors. It's also essential to have a sufficient amount of ETH in your wallet to pay for the deployment transaction.
Steps for Deploying Your Memecoin Contract
- Set up Your Ethereum Wallet: Use a wallet such as MetaMask to interact with the Ethereum network. Ensure it's funded with ETH to cover gas fees.
- Connect to a Deployment Tool: Use tools like Remix, Hardhat, or Truffle to compile and deploy your smart contract. Remix is particularly user-friendly for beginners.
- Compile the Smart Contract: In Remix, for example, you will need to compile the contract into bytecode. Ensure that no errors are present before proceeding.
- Deploy the Contract: After compilation, you can deploy the contract directly to the Ethereum mainnet or a testnet like Rinkeby for testing purposes.
- Confirm the Deployment: Once the transaction is complete, verify that the contract is successfully deployed by checking the contract address on Etherscan.
Important: Always test your contract on a testnet before deploying it on the mainnet to ensure everything works as expected and save on costly mistakes.
Gas Fees and Deployment Costs
Deploying a smart contract on the Ethereum network requires a fee called gas. Gas is needed to process and validate transactions, and its cost depends on the current network congestion. It’s important to monitor gas prices to avoid overpaying.
Gas Limit | Approximate Cost (ETH) | Remarks |
---|---|---|
150,000 - 200,000 | 0.02 - 0.05 ETH | Average cost for deploying a simple ERC-20 token contract |
300,000 - 400,000 | 0.05 - 0.1 ETH | For more complex smart contracts |
Tip: To optimize costs, try to deploy during times of lower network activity, which can help reduce gas fees.
Testing Your Meme Token on Ethereum's Testnet
Before deploying your meme coin on the Ethereum mainnet, it is crucial to test it on a test network to ensure its functionality. Ethereum provides several testnets, such as Rinkeby, Ropsten, and Goerli, where you can deploy your token without using real ETH. This process allows you to simulate real-world conditions and catch any potential issues before launching on the mainnet.
Testing on a testnet involves deploying the smart contract and interacting with it through decentralized applications (dApps) to verify its behavior. You'll need test ETH for transactions and gas fees, which can be obtained from faucet services. The following steps outline the key actions in testing your meme coin on Ethereum's test network.
Steps for Testing Your Meme Coin
- Deploy Your Smart Contract: Deploy the token smart contract on a testnet. Tools like Truffle, Hardhat, or Remix can be used for deployment.
- Fund Your Wallet: Obtain test ETH from a faucet to fund your wallet for gas fees and transactions.
- Verify Contract Behavior: Interact with the deployed contract to ensure it behaves as expected. Test transfers, minting, and other functions.
- Audit and Debug: Conduct code audits and debug any issues that arise during testing to avoid problems during the mainnet launch.
Key Points to Remember
- Test ETH: Use faucet services to get free ETH for testing.
- Gas Fees: Ensure the test ETH you have is sufficient to cover gas fees during testing.
- Security: Even on the testnet, ensure the security of your contract to avoid vulnerabilities.
Testing your meme coin thoroughly on a testnet is essential to avoid costly mistakes on the Ethereum mainnet. Make sure to verify all functions and resolve any issues early on.
Testing Results Overview
Test Activity | Status | Comments |
---|---|---|
Deploy Contract | Success | Contract deployed successfully on Rinkeby testnet. |
Mint Tokens | Success | Token minting worked as expected. |
Transfer Tokens | Pending | Need to adjust gas fee calculations. |
Creating a Wallet and Listing Your Meme Coin on Decentralized Exchanges
Once your meme coin is ready, the next steps involve ensuring it can be safely stored and traded. This requires creating a digital wallet to hold the coin and listing it on decentralized exchanges (DEXs) for liquidity. Both actions are essential for the practical use of your coin in the broader crypto ecosystem.
The process of creating a wallet is straightforward, and there are several options to choose from. Afterward, listing your coin on DEXs will allow potential buyers to exchange it for other cryptocurrencies. Here’s a breakdown of these processes.
Creating a Wallet for Your Meme Coin
The first step is to create a secure wallet for your meme coin. There are various wallet types, but the most common are software wallets and hardware wallets. The wallet will store your coin’s private keys, which are required for transactions.
- Software Wallets: Examples include MetaMask, Trust Wallet, and MyEtherWallet.
- Hardware Wallets: Options like Ledger and Trezor offer higher security for long-term storage.
- Paper Wallets: For those who prefer offline storage, a paper wallet can be created for added security.
Once the wallet is created, make sure to store the private key or recovery phrase in a safe place, as losing it will result in the permanent loss of access to your meme coin.
Listing Your Meme Coin on Decentralized Exchanges
After setting up a wallet, the next step is to list your meme coin on decentralized exchanges (DEXs). DEXs like Uniswap, SushiSwap, and PancakeSwap allow users to trade tokens directly from their wallets without relying on centralized authorities.
- Create a Liquidity Pool: You’ll need to add liquidity for your coin to facilitate trading. This often involves pairing your meme coin with a popular token like ETH or USDT.
- Deploy the Coin on a DEX: To list your coin, deploy it on a platform like Uniswap by providing the necessary contract details and liquidity pool settings.
- Get Listed on Aggregators: After listing, make sure your meme coin is included in token aggregators like CoinGecko and CoinMarketCap to increase visibility.
Ensure you comply with all the DEX’s listing requirements, which may include transaction fees and a minimum liquidity threshold.
Checklist for Listing Your Coin
Step | Action |
---|---|
1 | Create a Wallet |
2 | Generate Token Contract |
3 | Add Liquidity to DEX |
4 | Deploy Token |
5 | List on Token Aggregators |
Building a Community for Your Meme Coin: Marketing Strategies
Creating a meme coin is just the beginning. The next crucial step is building a community that supports and promotes your project. Strong community engagement is essential to generate hype, increase awareness, and drive value. To achieve this, you need to implement effective marketing strategies that resonate with potential investors and users.
Successful meme coin communities thrive on enthusiasm and participation. Your marketing efforts should encourage interaction, spark conversations, and make people feel involved. The more engaged your community, the more likely they are to spread the word and create organic growth. Below are some actionable strategies to help build a strong and loyal community.
Key Strategies for Growing Your Meme Coin Community
- Leverage Social Media: Utilize platforms like Twitter, Reddit, and Telegram to engage with potential users and share updates. Create dedicated channels where followers can interact directly with the project team.
- Influencer Partnerships: Collaborate with influencers in the crypto and meme space to expand your reach. Influencers can help you tap into established audiences and drive attention to your coin.
- Host Events and Giveaways: Organize online events, AMAs, or meme creation contests. Offering rewards such as free tokens can incentivize participation and keep the community active.
- Foster Transparency: Consistently share updates and progress on the project's development. Transparency builds trust and loyalty within your community.
How to Maintain Community Engagement
- Active Communication: Respond to community queries and feedback promptly. Build a reputation for accessibility and transparency.
- Gamification: Introduce challenges and incentives for community members to stay involved. This could include leaderboard systems or rewards for top contributors.
- Regular Content Updates: Keep the community excited by sharing fresh content, including memes, polls, and behind-the-scenes development stories.
Important: Community growth requires consistent effort. Success doesn’t happen overnight, so ensure your marketing efforts are ongoing and adaptable to changing trends in the crypto space.
Metrics for Measuring Community Growth
Metric | How to Track |
---|---|
Community Engagement | Monitor social media interactions, comments, and shares. |
Growth Rate | Track the number of new members joining your channels over time. |
Content Virality | Measure how often memes or content related to your coin is shared. |